Code Reference¶
Common¶
Common.Simulations¶
A placeholder for Simulation in the Common module. This module gather all simulations common things for TwoD, ThreeD, TwoDShape, models (and maybe others added afterwards).
- class Common.Simulations.GenericSimulation(terrain=None, gravity=9.8, name=None, project=None, enable_forest=False, random_seed=None)[source]¶
Bases:
object
Generic
Simulation
, parent of all simulations from PlatRock main modules (TwoD, ThreeD, TwoDShape, …). It contains common attributes and functions. ASimulation
object contains all informations concerning PlatRock simulations. Its child type defines the simulation model, its attributes stores the simulation setup and outputs. The run() method is used to launch the simulation (loops on rocks) via a set of generic methods.- Parameters:
random_seed (int, optional) – if given the random generator will be sed with this value, making simulation reproductible.
- name¶
the simulation name, useful for GUIs
- Type:
string
- terrain¶
binds a terrain to this simulation, must be compatible with the
Simulation
type.- Type:
compatible
Terrain
- gravity¶
positive gravity value
- Type:
float
- project¶
the project that contains this simulation, useful for GUIs
- Type:
Common.Projects.Project
- enable_forest¶
whether to enable the forest or not
- Type:
bool
- status¶
reflects the simulation state, will be modified internally
- Type:
string
- use_retro_compatibility¶
whether this simulation was loaded by
RetroCompatibility
or not- Type:
bool
- forest_impact_model¶
a bounce model that will handle rock-tree contacts, defaults to
Common.BounceModels.Toe_Tree_2022
- Type:
Common.BounceModels
subclass
- nb_rocks¶
how many rocks to launch, will be set internally
- Type:
int
- current_rock¶
points to the current simulated rock, will be set internally
- Type:
compatible
Rock
- benchmark¶
whether to enable yappi profiling tool or not
- Type:
bool
- checkpoints¶
the list of compatible checkpoints, will be set internally
- Type:
list of compatible
Checkpoint
- output¶
binds to the output instance, useful to retrieve simulation results
- Type:
- current_rock_number¶
how many rocks have already propagated, will be set internally
- Type:
int
- random_generator¶
set internally
- Type:
numpy.random.RandomState
- override_forest_params¶
whether to globally override all forest parameters or not
- Type:
bool
- override_rebound_params¶
whether to globally override all soil parameters or not
- Type:
bool
- override_[param_name]¶
where [param_name] are the soil/forest parameter to override (ex: override_R_n)
- Type:
various
- abort()[source]¶
If this simulation is launched via a GUI, this will abord its subprocess according to the process name, which sould be set to
get_full_path()
.
- after_rock_propagation_tasks(queue=None)[source]¶
Task method called after each single rock propagation is finished.
- after_successful_run_tasks()[source]¶
Task method called after all the rocks are propagated, if everything went right.
- get_dir()[source]¶
Get the path corresponding to the simulation, its the basepath of setup.json.
- Returns:
string
- get_full_path()[source]¶
Get the full path of the simulation pickle file, composed of: “/the_project_path/simulation_name/setup.json”
- Returns:
string
- rock_propagation_tasks()[source]¶
Here is the science, this task method must do the propagation main loops for a single rock.
- run(GUI=False, queue=None)[source]¶
Actually launch the simulation, contains the main loop on rocks and the calls to all other task methods.
- Parameters:
GUI (bool) – enables the ThreeD 3D view
queue (
multiprocessing.Queue
, optional) – this queue will be passed to other methods to communicate.
- save_to_file()[source]¶
This method will write the simulation into a json file located at
get_full_path()
with jsonpickle.Note
This method is not intented to be used as it, rather call it from the simulation subclass as we must before remove the unwanted heavy attributes.
Common.BounceModels¶
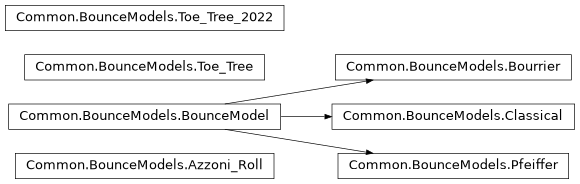
This module is the placeholder for all BounceModel
of PlatRock physical models. It is used by TwoD and ThreeD (non-siconos).
- class Common.BounceModels.Azzoni_Roll(vel, mu_r, slope, gravity, start_pos=None, tan_slope=None, A=None, mass=None, radius=None, I=None)[source]¶
Bases:
object
Class capable of performing rocks roll on slopes, based on [Azzoni 1995]. The constructor will compute the stop distance (and optionally the stop position) on a supposed infinite slope. After that, you can retrieve the rock velocity at a given position with
get_vel()
.- Parameters:
- vel¶
the input rock velocity
- Type:
Vector2
- mu_r¶
the rolling friction, this is tan_roll_phi in Azzoni paper
- Type:
float
- slope¶
the slope in radians
- Type:
float
- gravity¶
the gravity
- Type:
float
- start_pos¶
the rock input position, to compute the rock final position
- Type:
Vector2
, optional
- tan_slope¶
stores the tangent of the slope, use in constructor only if you don’t want the code to compute it from the slope
- Type:
float, optional
- A¶
you can manually set this intermediate result, so you don’t have to give the rock
mass
,radius
andI
(see [Azzoni 1995]).- Type:
float, optional
- delta_tan_angles¶
the difference between the two tangents of angles (slope and mu).
- Type:
float
- dist_stop¶
the resulting distance at which the rock will stop
- Type:
float
- stop_pos¶
the resulting position of the rock, if start_pos is given
- Type:
Vector2
- direction¶
stores the rock velocity direction along x: -1 or +1
- Type:
int
- v_square¶
stores the square of the rock velocity norm
- Type:
float
- compute_dist()[source]¶
Computes the distance at which the rock will stop if it were on an infinite slope, and store it into
dist_stop
. Ifstart_pos
is set, also compute thestop_pos
.
- get_vel(arrival_point)[source]¶
Get the arrival velocity from an initiated instance of
Azzoni_Roll
and an arrival point.- Parameters:
arrival_point (
Vector2
) – the precomputed arrival point- Returns:
the arrival velocity
- Return type:
Vector2
- class Common.BounceModels.BounceModel(simulation=None)[source]¶
Bases:
object
The parent class of every rock-soil bounce models. The table below represents the bounce-models dependency to the soil parameters. The soil parameters of the terrain elements (segments or faces) must be set according to this table. Similarly, the input files (csv or geojson) must be consistent with this table. Note that the parameters are case-sensitive.
bounce_model_number
roughness
mu_r
R_n
R_t
v_half
phi
Classical
Pfeiffer
Bourrier
- updated_normal¶
the segment (2D) or face (3D) rotated normal used to emulate roughness.
- Type:
Vector2
||Vector3
- simulation¶
the parent simulation, as the bounce models needs it to get its type and random generator. It should be a child class of
GenericSimulation
.- Type:
a child of
GenericSimulation
, optional
- check_output_vel_2D(r, f)[source]¶
Checks whether the rock velocity after rebound is valid, and the rocks doens’t go down inside the terrain.
- Parameters:
r (
TwoD.Objects.Rock
) – the current rockf (
TwoD.Objects.Segment
) – the segment on which the rock bounces
- get_velocity_decomposed(v)[source]¶
From the rock velocity in GCS, get the decomposed velocity in local CS (\(v_{n}\), \(v_t\)).
- Parameters:
v (
Vector2
||Vector3
) – input velocity- Returns:
the normal and tangential velocities in local CS.
- Return type:
list[\(v_n\), \(v_t\)]
- set_updated_normal(*args, **kwargs)[source]¶
Set
updated_normal
from the soil element normal, the rock velocity and the soil roughness.Note
This method is self-overriden by
set_updated_normal2D()
orset_updated_normal3D()
at its first call, depending on the simulation type. On previous implementations the binding was made in “__init__” but it caused jsonpickle to don’t load “set_updated_normal” function at all. Reason:Jsonpickle doesn’t run __init__ on load, but rather : - restore attributes from json file - “restore” methods from the existing class in memorySo in the previous implementation “set_updated_normal” was not an attribute, and neither a member function of BounceModel.
- Parameters:
*args – see
set_updated_normal2D()
orset_updated_normal3D()
**kwargs – see
set_updated_normal2D()
orset_updated_normal3D()
- set_updated_normal2D(rock, normal, roughness)[source]¶
Set
updated_normal
from the segment normal, the rock propagation direction and the soil roughness.- Parameters:
rock (
Rock
) – the current rocknormal (
Vector2
) – the current segment normalroughness (float) – the current segment roughness
- set_updated_normal3D(rock, normal, roughness)[source]¶
Set
updated_normal
from the face normal, the rock propagation direction and the soil roughness.- Parameters:
rock (
Rock
) – the current rocknormal (
Vector3
) – the current segment normalroughness (float) – the current segment roughness
- valid_input_attrs = <platrock.Common.Utils.ParametersDescriptorsSet object>¶
The set of parameters needed by all bounce models.
- class Common.BounceModels.Bourrier(*args)[source]¶
Bases:
BounceModel
Bourrier bounce model type (see [Bourrier2011]).
- valid_input_attrs = <platrock.Common.Utils.ParametersDescriptorsSet object>¶
The set of parameters needed by the Bourrier bounce model.
- class Common.BounceModels.Classical(*args)[source]¶
Bases:
BounceModel
Classical \(R_n, R_t\) bounce model type.
- run(r, f, disable_roughness=False)[source]¶
Runs the bounce model:
randomly compute the local slope angle
compute the rock velocity in the local slope coordinate system
apply \(R_n\) and \(R_t\) to the local velocity
finally rotate back the velocity into the global coordinates and set it to the rock.
- Parameters:
r (
TwoD.Objects.Rock
||ThreeD.Objects.Rock
) – the rock that bouncesf (
TwoD.Objects.Segment
||ThreeD.Objects.Face
) – the terrain element on which the rock bouncesdisable_roughness (bool, optional) – disables the
set_updated_normal()
function
- valid_input_attrs = <platrock.Common.Utils.ParametersDescriptorsSet object>¶
The set of parameters needed by the Classical bounce model.
- class Common.BounceModels.Pfeiffer(*args)[source]¶
Bases:
BounceModel
Pfeiffer bounce model type (see [Pfeiffer1989]).
- run(r, f, disable_roughness=False)[source]¶
Runs the bounce model:
randomly compute the local slope angle
compute the rock velocity in the local slope coordinate system
compute the friction function and the scaling factor
modify the velocity
finally rotate back the velocity into the global coordinates and set it to the rock.
- Parameters:
r (
TwoD.Objects.Rock
||ThreeD.Objects.Rock
) – the rock that bouncesf (
TwoD.Objects.Segment
||ThreeD.Objects.Face
) – the terrain element on which the rock bouncesdisable_roughness (bool, optional) – disables the
set_updated_normal()
function
- valid_input_attrs = <platrock.Common.Utils.ParametersDescriptorsSet object>¶
The set of parameters needed by the Pfeiffer bounce model.
- class Common.BounceModels.Toe_Tree[source]¶
Bases:
object
- get_output_vel(vel=None, vol=None, ecc=None, dbh=None, ang=None)[source]¶
Search in the abacus data array and retuns the nearest value.
- Parameters:
vel (float) – the input rock velocity norm
vol (float) – the volume of the rock
ecc (float) – the input rock eccentricity
dbh (float) – the tree diameter
ang (float) – the angle formed by the rock velocity and the horizontal plane
- Returns:
the rock output velocities \(v_x, v_y, v_z\).
- Return type:
numpy.ndarray
- valid_input_attrs = <platrock.Common.Utils.ParametersDescriptorsSet object>¶
The set of parameters needed by the Toe rock-tree bounce model.
- input_sequence¶
the ordered list of parameters in Toe dem abacus output
- Type:
list
- weight_sequence¶
the priority of the parameters when searching a value in the abacus, higher to lower
- Type:
list
- toe_array¶
stores the abacus data in a numpy array for fast multi-dimensional search
- Type:
numpy.ndarray
- last_computed_data¶
stores the last computed output properties for access from the outside
- Type:
dict
- class Common.BounceModels.Toe_Tree_2022[source]¶
Bases:
object
- cKDTree = None¶
- conv_tab = None¶
- data_file_url = 'https://entrepot.recherche.data.gouv.fr/api/access/datafile/:persistentId?persistentId=doi:10.57745/FY4IZI'¶
- max_dist = 10000¶
- params_weights = [1, 1, 1, 1, 1, 1]¶
- raw_data = None¶
- raw_data_col_offset = 1¶
- valid_input_attrs = <platrock.Common.Utils.ParametersDescriptorsSet object>¶
The set of parameters needed by the Toe rock-tree bounce model.
- Common.BounceModels.number_to_model_correspondance = {0: <class 'Common.BounceModels.Classical'>, 1: <class 'Common.BounceModels.Pfeiffer'>, 2: <class 'Common.BounceModels.Bourrier'>}¶
A dictionnary that allows to link between the rebound model numbers and the corresponding class.
Common.Outputs¶

This module is used to store output data in an optimized hdf5 file.
- Common.Outputs.FLY = 7¶
For
ThreeD.Postprocessings.Postprocessing
only, to describe a fly-over-raster-cell
- Common.Outputs.MOTION = 8¶
For
ShapedSimulations
only, to track the motion of the rocks (don’t really track contacts)
- Common.Outputs.OUT = 6¶
Rock out of terrain
- class Common.Outputs.Output(s)[source]¶
Bases:
object
Captures rocks contacts data for a whole simulation and organize them in a structured way. Getters functions helps to retrieve and select data from the strucure. Hdf5 file export is also supported.
- Parameters:
s (a
Simulation
instance) – will be_s
attribute.
- _s¶
binds to the simulation to retrieve informations from it.
- Type:
a
Simulation
instance
- contacts_slices¶
helper dict that links fields name to the corresponding columns in the
contacts
output array- Type:
dict of slices
- checkpoints¶
the list of checkpoints, binded to the simulations checkpoints.
- Type:
list of
checkpoint
- volumes¶
the 1D array of rocks volumes
- Type:
numpy.ndarray
- densities¶
the 1D array of rocks densities
- Type:
numpy.ndarray
- inertias¶
the 1D or 3D array of rocks inertias
- Type:
numpy.ndarray
- contacts¶
the list of contacts for all rocks, each rock is a numpy array with first dimension length equals the number of contacts, second dimension equals the number of fields recorded (see
contact_slices
).- Type:
list of
numpy.ndarray
- add_contact(r, normal, type_)[source]¶
Add a contact to the last rock added to the output. This is triggered by the
rock_propagation_tasks()
functions at each contact. It will store contact-related infos, such as position, velocity…- Parameters:
r (
Rock
)normal (
platrock.Common.Math.Vector2
|Common.Math.Vector3
) the contact normal (branch vector)type (int) – one of the contact type (ex:
SOIL
)
- add_rock(r)[source]¶
Add a rock with no contacts to the output. This is triggered by the
before_rock_launch_tasks()
functions. Stores rock quantities such a volume, density, inertia…- Parameters:
r (
Rock
) – instanciated rock
- get_contacts_angVels(rock_id)[source]¶
From a rock number, retrive all contacts angVels.
- Parameters:
rock_id (int) – the rock index in
contacts
from which to retrieve infos- Returns:
numpy.ndarray
- get_contacts_field(rock_id, field)[source]¶
From a rock number, retrive all contact data corresponding to the field name (see
contacts_slices
)- Parameters:
rock_id (int) – the rock index in
contacts
from which to retrieve infosfield (string) – the data to retrieve (see keys of
contacts_slices
)
- Returns:
numpy.ndarray
- get_contacts_normals(rock_id)[source]¶
From a rock number, retrive all contacts normals.
- Parameters:
rock_id (int) – the rock index in
contacts
from which to retrieve infos- Returns:
numpy.ndarray
- get_contacts_pos(rock_id)[source]¶
From a rock number, retrive all contacts positions.
- Parameters:
rock_id (int) – the rock index in
contacts
from which to retrieve infos- Returns:
numpy.ndarray
- get_contacts_types(rock_id)[source]¶
From a rock number, retrive all contacts types.
- Parameters:
rock_id (int) – the rock index in
contacts
from which to retrieve infos- Returns:
numpy.ndarray
- get_contacts_vels(rock_id)[source]¶
From a rock number, retrive all contacts velocities.
- Parameters:
rock_id (int) – the rock index in
contacts
from which to retrieve infos- Returns:
numpy.ndarray
- write_to_h5(filename)[source]¶
Export this output to a hdf5 file.
Note
It is advised to use hdf-compass to read hdf5 files. Use
sudo apt install hdf-compass
on debian-based distros such as Ubuntu.Note
Here is the hdf5 file structure created:
# hdf5-file/ # ├── rocks/ # | ├── start_data # | └── contacts/ # | ├── 0 (contacts of rock n°0) # | ├── .. # | └── nb_rocks # └── checkpoints/ # ├── x_0 (x position of checkpoint 0) # ├── x_1 # └── x_N
- Common.Outputs.ROLL = 4¶
Rolling-friction
- Common.Outputs.ROLL_TREE = 3¶
Contact with a tree while rolling
- Common.Outputs.SOIL = 1¶
Rock-soil contact
- Common.Outputs.START = 0¶
The rock initial position
- Common.Outputs.STOP = 5¶
Rock stop position
- Common.Outputs.TREE = 2¶
Rock-tree contact
Common.OneDTreeGenerator¶

Module that handles the generation of trees.
- class Common.TreesGenerators.OneDTreeGenerator(sim, treesDensity=0.1, trees_dhp=0.3, dRock=0.5)[source]¶
Bases:
object
Computes the (1D) distance before the next tree impact from the trees/rock properties and a probability law. Instanciate then launch
getOneRandomTreeImpactDistance()
to get the next distance.Note
The cdf follow an exponential law which parameter is a function of basal area (treesDensity*trees_dhp), trees_dhp and dRock.
- sim¶
the parent simulation
- Type:
GenericSimulation
, optional
- treesDensity¶
the trees density, in trees/m²
- Type:
float
- trees_dhp¶
the trees mean diameter, in meters
- Type:
float, optional
- dRock¶
the rock equivalent diameter, in meters
- Type:
float, optional
- coef¶
the computed parameters of the cdf exponential law
- Type:
float
- prob0¶
the probability to hit a tree immediately
- Type:
float
- random_generator¶
copied from simulation or
numpy.random
Common.Math¶
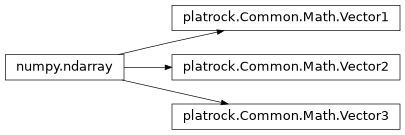
Cython module intented to do mathematical operations as fast as possible.
- class platrock.Common.Math.Vector1(input=0.0)¶
Bases:
ndarray
Mainly for defining angular velocity in 2D models, and still allows for computations while sharing bounce models between 2D and 3D models. Instanciation follows numpy.ndarray directives.
- class platrock.Common.Math.Vector2(input=[0, 0])¶
Bases:
ndarray
Two components vector with fast operations, also allows for computations while sharing bounce models between 2D and 3D models. Instanciation follows numpy.ndarray directives.
- class platrock.Common.Math.Vector3(input=[0, 0, 0])¶
Bases:
ndarray
3 components vector with fast operations, also allows for computations while sharing bounce models between 2D and 3D models. Instanciation follows numpy.ndarray directives.
- platrock.Common.Math.atan2_unsigned(y, x)¶
Returns atan2, but shifted in the interval [\(0\), \(2\pi\)].
- Parameters:
y (float)
x (float)
- Returns:
float
- platrock.Common.Math.center_2d_polygon_vertices(points)¶
Move in place a 2D array of points forming a polygon to set its COG to [0,0].
- Parameters:
points (
numpy.ndarray
) – the array of points forming the polygon in the form [[x0, y0], [x1, y1], …]
- platrock.Common.Math.dot1(A, B)¶
Get the dot product of this
Vector1
by B, equivalent to regular scalar product.- Parameters:
B (
Vector1
)- Returns:
float
- platrock.Common.Math.dot2(A, B)¶
Get the dot product of this
Vector2
by B.- Parameters:
B (
Vector2
)- Returns:
float
- platrock.Common.Math.dot3(A, B)¶
Get the dot product of this
Vector3
by B.- Parameters:
B (
Vector3
)- Returns:
float
- platrock.Common.Math.get_2D_polygon_area_inertia(points, density, cog_centered=False)¶
From a 2D array of points forming a polygon, get its area and inertia.
- Parameters:
points (
numpy.ndarray
) – the array of points forming the polygon in the form [[x0, y0], [x1, y1], …]density (float) – the 2D polygon density, used to compute inertia
cog_centered (bool, optional) – whether the polygon COG in also at coordinates [0,0] or not.
- Returns:
the area and inertia, \([A, I]\).
- Return type:
list
- platrock.Common.Math.get_2D_polygon_center_of_mass(points)¶
From a 2D array of points forming a polygon, get its center of mass.
Note
- Parameters:
points (
numpy.ndarray
) – the array of points forming the polygon in the form [[x0, y0], [x1, y1], …]- Returns:
the center of mass coordinates \([G_x, G_y]\)
- Return type:
list
- platrock.Common.Math.get_random_convex_polygon(n, Lx, Ly)¶
Get a random convex 2D array of points forming a polygon.
Note
Valtr’s algorithm is used, it can be found here : https://cglab.ca/~sander/misc/ConvexGeneration/convex.html
- Parameters:
n (int) – the number of vertices
Lx (float) – the polygon size in the \(x\) direction
Ly (float) – the polygon size in the \(y\) direction
- Returns:
2D array of points forming the polygon
- Return type:
numpy.ndarray
- platrock.Common.Math.get_random_value_from_gamma_distribution(mean, std, rand_gen=None)¶
Get a random value from a given gamma distribution. Uses scipy.stats.
- Parameters:
mean (float) – the desired distribution mean
std (float) – the desired distribution standard deviation
rand_gen (
numpy.random.RandomState
, optional) – the random generator, useful for simulations reproductibility.
- Returns:
float
- platrock.Common.Math.norm1(A)¶
Get the norm of this
Vector1
, equivalent to absolute value.- Returns:
float
- platrock.Common.Math.rotate_points_around_origin(points, radians)¶
Rotate in place a 2D array of points around [0,0].
- Parameters:
points (
numpy.ndarray
) – the array of points in the form [[x0, y0], [x1, y1], …]radians (float) – the amount of rotation, (angle in radians)
- platrock.Common.Math.rotate_vector(q, A)¶
Get a rotated quaternion from an input quaternion q with a rotation vector A.
- Parameters:
q (
quaternion
) – the quaternion to turnA (
Vector3
) – the rotation vector
- Returns:
quaternion
- platrock.Common.Math.sort_2d_polygon_vertices(points)¶
Sort in place a 2D array of points to form a polygon.
- Parameters:
points (
numpy.ndarray
) – the array of points in the form [[x0, y0], [x1, y1], …]
Common.Utils¶
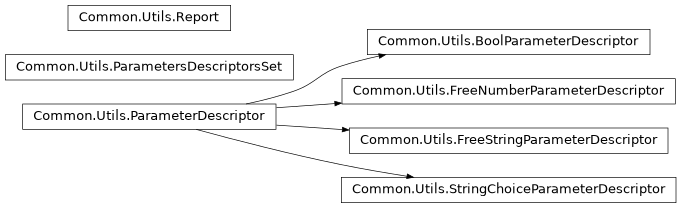
Some handy classes and functions used everywhere in PlatRock. FIXME: DOC
- class Common.Utils.BoolParameterDescriptor(input_names, inst_name, human_name, default_value=None)[source]¶
Bases:
ParameterDescriptor
- TYPE = 'Bool'¶
- class Common.Utils.FreeNumberParameterDescriptor(input_names, inst_name, human_name, type_, min_value=None, max_value=None, default_value=None)[source]¶
Bases:
ParameterDescriptor
- TYPE = 'FreeNumber'¶
- class Common.Utils.FreeStringParameterDescriptor(input_names, inst_name, human_name, default_value='', hint=False)[source]¶
Bases:
ParameterDescriptor
- TYPE = 'FreeString'¶
- class Common.Utils.ParameterDescriptor(input_names, inst_name, human_name, type_, default_value)[source]¶
Bases:
object
Fully describes a parameter, useful for GUIs.
Note
This class doesn’t contain the value of the parameter, only its characteristics. Values are directly stored in instances,
GenericSimulation
for example.- input_names¶
when reading platrock input parameters names from files, use this(ese) name(s) to bind to the right parameter. Always store as a list of strings in instances.
- Type:
list of strings || string
- inst_name¶
the instance name, name that this parameter will take in platrock source code, objects instances, etc…
- Type:
string
- human_name¶
a human-readable and understandable full parameter name, including eventual unity
- Type:
string
- type_¶
the python type the parameter should be of
- Type:
type
- set_to_obj(obj, value=None, inst_name_prefix='', inst_name_suffix='')[source]¶
Set a value from this parameter descriptor to an object attribute. This will cast to
type_
and set thedefault_value
if the value given is None. The attribute name will beinst_name
.- Parameters:
obj (object) – the instance to set the parameter to
value (anything castable to
type_
, optional) – the value to set, if Nonedefault_value
will be usedinst_name_prefix (string, optional) – a prefix added before
inst_name
inst_name_suffix (string, optional) – a suffix added after
inst_name
- class Common.Utils.ParametersDescriptorsSet(params_list)[source]¶
Bases:
object
A container of
ParameterDescriptor
, with helper functions.Note
ParametersDescriptorsSet
are not iterable, useparameters
attribute to loop on containedParameterDescriptor
.- Parameters:
params_list (list of
ParameterDescriptor
|| list of list of args to constructParameterDescriptor
) – the input set ofParameterDescriptor
- parameters¶
contains the
ParameterDescriptor
- Type:
list
- instance_names_to_parameters¶
links
ParameterDescriptor.inst_name
toParameterDescriptor
- Type:
dict
- input_names_to_parameters¶
links
ParameterDescriptor.input_names
toParameterDescriptor
- Type:
dict
- add_parameter(*args, **kwargs)[source]¶
Adds a
ParameterDescriptor
into this set, either by passing aParametersDescriptorsSet
or args/kwargs that satisfies theParameterDescriptor
constructor.:param A single
ParameterDescriptor
: :param or a set of arguments compatible withParameterDescriptor
constructor signature.:- Kwargs:
A set of keywords arguments compatible with
ParameterDescriptor
constructor signature.
- get_human_names()[source]¶
Get all the human names of the
ParameterDescriptor
contained in this container- Returns:
list of strings
- get_input_names()[source]¶
Get all the input names of the
ParameterDescriptor
contained in this container- Returns:
list of strings
- get_instance_names()[source]¶
Get all the instance names of the
ParameterDescriptor
contained in this container- Returns:
list of strings
- get_param_by_input_name(input_name)[source]¶
Get a
ParameterDescriptor
from its input name.- Parameters:
input_name (string) – the input name to search for
- Returns:
returns false if the param input name doesn’t exist.
- Return type:
ParameterDescriptor
|| bool
- get_param_by_instance_name(instance_name)[source]¶
Get a
ParameterDescriptor
from its instance name.- Parameters:
instance_name (string) – the instance name name to search for
- Returns:
returns false if the param instance name doesn’t exist.
- Return type:
ParameterDescriptor
|| bool
- class Common.Utils.Report[source]¶
Bases:
object
A report is a handy tool that groups a series of messages with corresponding message type. It is used to check users parameters in WebUI, before they launch a simulation.
- nb_of_errors¶
counts the number of errors contained is this report
- Type:
int
- messages¶
contains all the messages in the format [[mess_type, “the message”], …]
- Type:
list
- add_error(message)[source]¶
Adds an error (“E”) message to the report. Note that this will increment
nb_of_errors
.- Parameters:
message (string) – the message
- add_info(message)[source]¶
Adds an info (“I”) message to the report.
- Parameters:
message (string) – the message
- add_message(_type, message)[source]¶
Adds a message to the report, should not really be used directly.
- Parameters:
_type (sting) – one of the
messages_letters_to_types
keys (for example “I”)message (string) – the message
- add_warning(message)[source]¶
Adds an warning (“W”) message to the report.
- Parameters:
message (string) – the message
- check_parameter(param_descriptor, param_value, location_string='')[source]¶
Checks the validity of a value for a given
ParameterDescriptor
. The value type and range will be checked, if they are not valid some error messages will be added to this report.- Parameters:
param_descriptor (
ParameterDescriptor
) – the parameter descriptor containing the type and range for the valueparam_value (any) – the value to check
location_string (string) – for the error message to be more explicit, tell where the parameter comes from.
- messages_letters_to_types = {'E': 'ERROR', 'I': 'INFO', 'W': 'WARNING'}¶
Links between the message letter types (_type) and the corresponding human names
- class Common.Utils.StringChoiceParameterDescriptor(input_names, inst_name, human_name, default_value='', choices=[''])[source]¶
Bases:
ParameterDescriptor
- TYPE = 'StringChoice'¶
- Common.Utils.extract_geojson_to_params_set(filename, destination, validation_params_descriptors_set, colormap)[source]¶
Get geometries and parameters from a geojson file, and populate the
destination
list.Note
The
destination
should be an instanciated list, for example as an object attribute, as it will be cleared then modified in place. Its format will be:[ { "shapely_polygon": <the_corresponding_shapely_polygon_instance>, "params": { "R_n": 0.5, "R_t": 0.2, ... }, "color": [0.5, 0.5, 0.5] } ]
- Parameters:
filename (string) – path to the geojson file
destination (list) – points to a list that will contains the parameters and geometries
validation_params_descriptors_set (
ParametersDescriptorsSet
) – the parameters coming from the geojson file will be validated with this parameter containercolormap (list) – a (2D) list of rgb colors to set a “color” to each output parameter set
- Returns:
0 if extraction is OK, the error message otherwise
- Common.Utils.get_geojson_all_properties(filename, unique=True)[source]¶
Get all available “properties” names for all features of a geojson file.
- Parameters:
filename (string) – path to the geojson file
unique (bool, optional) – if set to False, all properties names of all features of the file will be output (2D list). If set to True the properties names of all features will be merged, but each parameter will appear once (1D list).
- Returns:
list of strings
- Common.Utils.get_geojson_all_shapes(filename, unique=True)[source]¶
Get all available “geometry” types for all features of a geojson file.
- Parameters:
filename (string) – path to the geojson file
unique (bool, optional) – if set to False, all geometry names of all features of the file will be output (2D list). If set to True the geometry names of all features will be merged, but each geometry will appear once (1D list).
- Returns:
list of strings
Common.Debug¶
This module is used by PlatRock for logging. Log level can be set with current_level or by launching platrock -ll=info (replace info by the log level you want). It is also used by the WebUI to log to files instead of stdout.
- Common.Debug.ERROR = 40¶
Only log errors
- Common.Debug.INFO = 20¶
The more verbose mode.
- Common.Debug.WARNING = 30¶
Intermediate verbosity.
- Common.Debug.add_logger(filename=None)[source]¶
Get or create a logger based on the process PID, clear its handlers, add a new handler.
- Parameters:
filename (string, optional) – the filename to write into, otherwise log will output to stdout.
- Common.Debug.args_to_str(*args)[source]¶
Concatenate stringified variables.
- Parameters:
*args (any) – the arguments to be stringified.
- Returns:
all args as strings, separated by spaces.
- Return type:
str
- Common.Debug.current_level = 0¶
Current log level. This is set by bin/platrock.
- Common.Debug.error(*args)[source]¶
Write variables to current logger if the current log level is superior or equal to
ERROR
.- Parameters:
*args (any) – the variables to log.
- Common.Debug.get_logger()[source]¶
Get the logger corresponding to the current process PID, or create one if it doesn’t exist (this is the behavior of “logging.getLogger”)
- Returns:
the python logger to write into.
- Return type:
logging.RootLogger
- Common.Debug.info(*args)[source]¶
Write variables to current logger if the current log level is superior or equal to
INFO
.- Parameters:
*args (any) – the variables to log.
- Common.Debug.init(level)[source]¶
This function sets the
current_level
and overrides theinfo()
,warning()
,error()
functions withdo_nothing()
depending on thelevel
.
TwoD¶
TwoD.Simulations¶
- class TwoD.Simulations.Simulation(*args, **kwargs)[source]¶
Bases:
GenericTwoDSimulation
A TwoD simulation.
Constructor
- Parameters:
checkpoints_x (list [x0, x1, ...]) – all the checkpoints x postions. Checkpoints data are post-computed with
update_checkpoints()
from all contacts of all rocks.
- rock_propagation_tasks()[source]¶
Here is the science, this task method must do the propagation main loops for a single rock.
- webui_typename = 'PlatRock 2D'¶
TwoD.Objects¶

This module handles the objects types needed by the TwoD model.
- class TwoD.Objects.Checkpoint(_x=None, angle=None, max_height=None)[source]¶
Bases:
GenericTwoDCheckpoint
- class TwoD.Objects.Rock(*args, **kwargs)[source]¶
Bases:
GenericTwoDRock
A falling rock.
- Parameters:
x (float) – initial position along the x axis. Note that the coordinates system used is the one after the terrain is eventually cleaned, shifted and reversed.
height (float) – initial height relative to the terrain
- vel¶
velocity along x,z
- Type:
Common.Math.Vector2
[float,float]
- angVel¶
angular velocity
- Type:
float
- volume¶
volume
- Type:
float
- density¶
density
- Type:
float
- I¶
inertia, autocomputed if not given
- Type:
float
- radius¶
radius, autocomputed
- Type:
float
- mass¶
mass, autocomputed
- Type:
float
- A¶
an intermediate result of the Azzoni Roll, automatically precomputed
- Type:
float
- v_square¶
an intermediate value computed into the Azzoni Roll
- Type:
float
- force_roll¶
a flag set/used into the roll algorithm to handle roll along two colinear segments
- Type:
bool
- is_stopped¶
flag triggered when the stopping condition is reached
- Type:
boolean
- flying_direction¶
-1 if the rock is moving towards -x, +1 if the rock is moving towards +x
- Type:
int
- color¶
the rock RGB color, each compound being between 0. and 1., autocomputed
- Type:
list [float,float,float]
- out_of_bounds¶
set to true during the simulation if the rock went out of the terrain
- Type:
bool
- bounce(s, segment, disable_roughness=False)[source]¶
Apply a bounce from
platrock.Common.BounceModels
to the rock, it will update the velocity and angVel of the rock.- Parameters:
s (
Simulation
) – the current simulation, needed to access its outputsegment (
Segment
) – the segment that is vertically under the rockdisable_roughness (bool) – use this to tell the bounce model not to apply the terrain roughness
- fly(arrival_point, s, segment)[source]¶
Apply a fly movement to the rock, it will update the position and the velocity of the rock.
- Parameters:
arrival_point (
Vector2
) – the new position along x,zs (
Simulation
) – the current simulation, needed to access its outputsegment (
Segment
) – the segment that is vertically under the rock after the move
- move(arrival_point, s, segment)[source]¶
Actually move the rock by updating its
pos
. The current simulation and arrival segment must be given as they are usually already computed at this stage.Note
This method also handles the rock stop condition, it has in charge to set
is_stopped
.- Parameters:
arrival_point (
Vector2
) – the new position along x,zs (
Simulation
) – the current simulation, needed to access its outputsegment (
Segment
) – the segment that is vertically under the rock after the move
- roll(s, azzoni_roll, arrival_point)[source]¶
Apply a roll movement to the rock, it will update the position, velocity and angVel of the rock.
- Parameters:
s (
Simulation
) – the current simulation, needed to access its outputazzoni_roll (
Azzoni_Roll
) – the instanciated roll model as it contains necessary attributes and methodsarrival_point (
Vector2
) – the new position along x,z
- update_flying_direction()[source]¶
Deduce and update the
flying_direction
from the velocity.
- class TwoD.Objects.Segment(start_point, end_point)[source]¶
Bases:
GenericSegment
A segment of the terrain. Attributes from all bounce models / rolls will be considered as valid, they are stored in
valid_input_attrs
, with values :instance name
input name(s)
human name
roughness
roughness
Roughness
bounce_model_number
bounce_model_number || bounce_mod
Rebound model
mu_r
mu_r
μ \(_{r}\)
R_t
R_t
R \(_{t}\)
R_n
R_n
R \(_{n}\)
phi
phi
φ
v_half
v_half
V \(_{1/2}\)
trees_density
trees_density || density
Trees density (trees/ha)
trees_dhp_mean
trees_dhp_mean || dhp_mean
Mean trees ⌀ (cm)
trees_dhp_std
trees_dhp_std || dhp_std
σ(trees ⌀) (cm)
- Parameters:
- points¶
start and end points in the form \([ [x_{start}, z_{start}], [x_{end}, z_{end}] ]\)
- Type:
np.ndarray
- index¶
index of the segment (they are continuously and automatically indexed through the terrain)
- Type:
int
- slope_gradient¶
the gradient of slope (\(\Delta_z / \Delta_x\))
- Type:
float
- slope¶
the slope in radians, CCW
- Type:
float
- valid_input_attrs = <platrock.Common.Utils.ParametersDescriptorsSet object>¶
Describes the available soil attributes, they are a concatenation of all bounce models parameters.
- class TwoD.Objects.Terrain(*args, **kwargs)[source]¶
Bases:
GenericTwoDTerrain
A 2D terrain made of segments.
- Parameters:
file (string)
- rebound_models_available¶
A list of available bounce models numbers regarding the per-segment input parameters given, automatically filled.
- Type:
list
- forest_available¶
whether the forest is available in the terrain regarding the per-segment input parameters given, automatically set.
- Type:
bool
- check_segments_parameters_consistency()[source]¶
Analyze the segments parameters and checks their consistency/availability.
forest_available
andrebound_models_available
are here.
- valid_input_attrs = <platrock.Common.Utils.ParametersDescriptorsSet object>¶
TwoD.Geoms¶
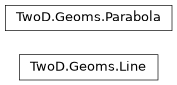
This module is used by the TwoD model. It describes 2D geometric objects and provide some functions to solve geometric problems.
- class TwoD.Geoms.Line(S=None, a=None, b=None, point=None, angle=None)[source]¶
Bases:
object
A line, described by the two constants
a
andb
in \(z=ax + b\). The instanciation can be done with either from:a segment S
a and b constants
[x, y] of a point and a positive angle in radians
- Parameters:
S (
TwoD.Objects.Segment
, optional) – a segment from which to deduce the line.
- a¶
the rate of increase coefficient.
- Type:
float
- b¶
the y-intercept.
- Type:
float
- EPS = 1e-10¶
- class TwoD.Geoms.Parabola(r=None, pos=None, vel=None, g=None, ABC=None)[source]¶
Bases:
object
A 2D parabola, described by : \(z = Ax^2 + Bx +C\). The instanciation can be done with either from:
\(g\) and the velocity and position of a
TwoD.Objects.Rock
,\(g\) and an explicit pair of position and velocity,
directly from an ABC vector/list.
- Parameters:
r (
TwoD.Objects.Rock
, optional) – a rock from which to deduce the parabolapos (iterable, optional) – a position in a list, a vector or an array \([px, pz]\)
vel (iterable, optional) – a velocity in a list, a vector or an array \([vx, pz]\)
g (float, optional) – the gravity acceleration
ABC (iterable, optional) – the A, B and C constants in a list, a vector or an array \([A, B, C]\)
- A¶
the \(A\) square parameter
- Type:
float
- B¶
the \(B\) linear parameter
- Type:
float
- C¶
the \(C\) constant parameter
- Type:
float
- get_arrow_x_from_gradient(gradient)[source]¶
Returns the \(x\) value of the arrow for this parabola and a slope defined by its gradient. If the parabola is a trajectory, the arrow is the highest (vertical) height of the object during its fly over the slope.
- Parameters:
gradient (float) – the slope gradient (\(dx/dy\))
- Returns:
\(x_{arrow}\)
- Return type:
float
- TwoD.Geoms.find_next_bounce(sim, rock, rock_parabola)[source]¶
In a given simulation, for a given rock, find the next rebound on the soil. The algorithm works as follows:
compute the rock parabola
loop on the segments from the
TwoD.Objects.Rock.current_segment
in theTwoD.Objects.Rock.flying_direction
, and try to find a parabola-segment intersectionkeep the intersection with the soil if it is compatible with the rock flying direction and if it does not correspond to the previous rock rebound point
- Parameters:
sim (
TwoD.Simulations.Simulation
) – the simulationrock (
TwoD.Objects.Rock
) – the rock
- Returns:
(intersection point, corresponding segment)
- Return type:
(
Vector2
[x_i, z_i]
,TwoD.Objects.Segment
)
- TwoD.Geoms.get_line_parabola_intersections(L, P)[source]¶
Computes the intersections between a line and a parabola. If no solutions, return a NaN-filled array.
- Parameters:
L (
TwoD.Geoms.Line
) – the lineP (
TwoD.Geoms.Parabola
) – the parabola
- Returns:
the two solutions
[[x_i1,z_i1], [x_i2, z_i2]]
:- Return type:
numpy.ndarray
- TwoD.Geoms.get_roots(A, B, C)[source]¶
Gives the roots of the second order equation \(z=Ax^2 + Bx + C\). If there is no solutions, the returned array is empty. If the there is one solution only, it will be duplicated in the output array.
- Parameters:
A (float) – the square coefficient
B (float) – the linear coefficient
C (float) – the constant coefficient
- Returns:
array containing the two roots \([x_{root1}, x_{root2}]\), or empty array.
- Return type:
- TwoD.Geoms.get_segment_parabola_intersections(S, P)[source]¶
Computes the intersection(s) between a segment and a parabola. If no solutions, return an empty array.
- Parameters:
S (
TwoD.Objects.Segment
) – the segmentP (
TwoD.Geoms.Parabola
) – the parabola
- Returns:
the eventual solutions
[[x_i1, z_i1], [x_i2, z_i2]]
.- Return type:
numpy.ndarray
TwoDShape¶
TwoDShape.Simulations¶
TwoDShape.Objects¶

- class TwoDShape.Objects.Checkpoint(_x=None, angle=None, max_height=None)[source]¶
Bases:
GenericTwoDCheckpoint
- class TwoDShape.Objects.Ellipse(**kwargs)[source]¶
Bases:
Rock
- valid_shape_params = <platrock.Common.Utils.ParametersDescriptorsSet object>¶
- class TwoDShape.Objects.PointsList(points=None, **kwargs)[source]¶
Bases:
Rock
- static input_string_to_points(input_str)[source]¶
NOTE: input_str can be an xy filepath or a string representing the content of an xy file.
- valid_shape_params = <platrock.Common.Utils.ParametersDescriptorsSet object>¶
- class TwoDShape.Objects.Random(**kwargs)[source]¶
Bases:
Rock
- valid_shape_params = <platrock.Common.Utils.ParametersDescriptorsSet object>¶
- class TwoDShape.Objects.Rectangle(**kwargs)[source]¶
Bases:
Rock
- valid_shape_params = <platrock.Common.Utils.ParametersDescriptorsSet object>¶
- class TwoDShape.Objects.Rock(**kwargs)[source]¶
Bases:
GenericTwoDRock
- setup_kinematics(ori=None, **kwargs)[source]¶
Set the rock kinematics quantities, such as velocity and position.
- valid_shape_params = <platrock.Common.Utils.ParametersDescriptorsSet object>¶
ThreeD¶
ThreeD.Simulations¶
- class ThreeD.Simulations.Simulation(engines=None, dt=0.02, **kwargs)[source]¶
Bases:
GenericThreeDSimulation
,GenericTimeSteppedSimulation
A simulation
The table below displays the attributes that have to be set to the polygons of the input geojson rocks_start_params_geojson depending on the sub-model choosen.
number
z
volume
vz
length
width
height
PlatRock (builtin)
Siconos
- Parameters:
rocks (list [
ThreeD.Objects.Rock
]) – list of all rocks, created in the launching script (see Examples/3D.py)current_rock (
ThreeD.Objects.Rock
) – the rock currently fallingterrain (
ThreeD.Objects.Terrain
) – the terrain of the simulationgravity (float) – the — positive — gravity acceleration value
enable_forest (bool) – whether to take trees into account or not
engines (list [
ThreeD.Engines.Engine
]) – list of engines to run at each timestepdt (int) – the time-step
iter (int) – the current iteration (reseted at each new rock)
running (bool) – whether the simulation is running
enable_GUI (bool) – enables the 3D view (experimental)
- rock_propagation_tasks()[source]¶
Here is the science, this task method must do the propagation main loops for a single rock.
- valid_input_rocks_geojson_attrs = <platrock.Common.Utils.ParametersDescriptorsSet object>¶
- webui_typename = 'PlatRock 3D'¶
ThreeD.Objects¶
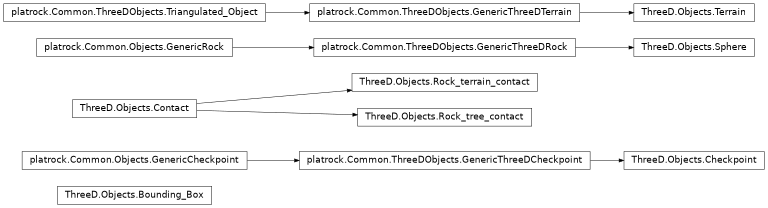
- class ThreeD.Objects.Terrain(default_faces_params={'R_n': 0.6, 'R_t': 0.6, 'bounce_model_number': 0, 'e': 0.1, 'mu': 0.8, 'mu_r': 0.5, 'phi': 30, 'roughness': 0.1, 'v_half': 2.5}, *args, **kwargs)[source]¶
Bases:
GenericThreeDTerrain
- bounce_class¶
alias of
Bourrier
- valid_input_soil_geojson_attrs = <platrock.Common.Utils.ParametersDescriptorsSet object>¶
ThreeD.Engines¶
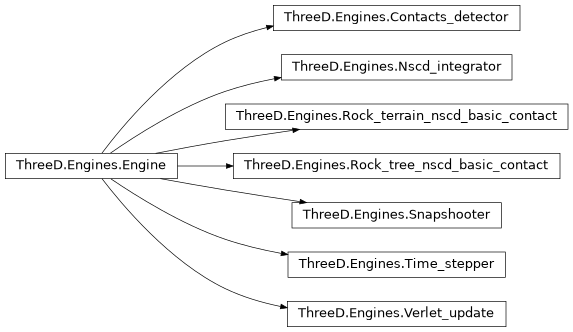
- class ThreeD.Engines.Contacts_detector(**kwargs)[source]¶
Bases:
Engine
FIXME:DOC Append (real, geometrical) contacts into
ThreeD.Objects.Rock.terrain_active_contacts
dict. Rock-Face goes intoterrain_active_contacts["terrain"]
and Rock-Tree contacts goes intoterrain_active_contacts["tree"]
.
- class ThreeD.Engines.Engine(iter_every=1, use_cython=True)[source]¶
Bases:
object
“Abstract” class for all engines. Each engine has a run() method which is run at each “iter_every” iteration. Engines instances must be stored in
ThreeD.Simulations.Simulation.Engines
.- Parameters:
dead (bool) – if True, the engine will not be ran
iter_every (int) – run the engine each iter_every timesteps
- class ThreeD.Engines.Verlet_update(dist_factor=5, **kwargs)[source]¶
Bases:
Engine
Update the rocks faces (
ThreeD.Objects.Rock.verlet_faces_list
) and trees (ThreeD.Objects.Rock.verlet_trees_list
) neighbours lists.- Parameters:
dist_factor (float) – the verlet distance factor (dist_factor * rock_radius = verlet distance). Values must be >1, but values >5 are highly recommanded.If dist_factor==1, the verlet algorithm will be unefficient.
Common.RasterTools¶

Common.ThreeDPostprocessings¶
